Step 1: Make it
What is it?
Turn your BBC micro:bit into a tool for measuring temperature, light and sound levels as part of a science investigation into your environment.
Introduction
Coding guide
How to use it
- Use this project to turn your micro:bit into a thermometer, and sound and light meters for taking measurements in science experiments.
- Transfer the code below to your micro:bit, or watch the coding video above if you want to create the code yourself.
- Attach a battery pack to your micro:bit and you’re ready to start.
- You can use our data recording sheet for recording your measurements.
Temperature
Use the micro:bit as a thermometer to measure differences in temperature in different places. Press button A to show the temperature in degrees Celsius on the display. It’s a good idea to leave the micro:bit in a new location for a couple of minutes to ensure you get an accurate reading.
Sound
To use the micro:bit as sound level meter, press button B to show the sound level on a scale from 0 (quietest) to 255 (loudest).
There’s a short delay before it takes the sound reading to make sure the sound of pressing the button isn’t recorded.
Light
To use the micro:bit as a light meter, press buttons A and B together. It shows light level readings, on a scale from 0 (darkest) to 255 (lightest).
Analyse your data
Top tip: gathering as much data as you can is good scientific practice, so you might want to take several readings in each location and calculate an average.
Once you’ve recorded your data you can analyse it to draw conclusions. What is there to learn from your data about temperature, sound and light levels around you?
Where was warmest, coolest, loudest, quietest, lightest, or darkest and what factors may have affected this?
What you need
- micro:bit
- MakeCode or Python editor
- battery pack
- data recording sheet, or other paper
- pen or pencil
Data recording sheet
The data recording sheet can be used to record your measurements.
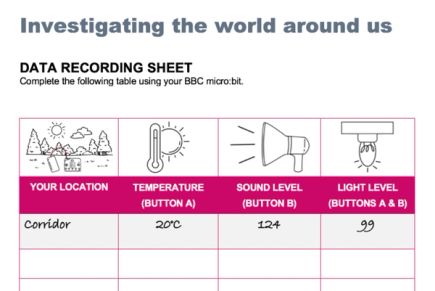
Step 2: Code it
1from microbit import *
2
3# The Python version of this project has sleep()
4# for each button press to make the A+B button work better
5
6while True:
7 if button_a.is_pressed() and button_b.is_pressed():
8 display.scroll(display.read_light_level())
9 sleep(200)
10 elif button_a.is_pressed():
11 display.scroll(temperature())
12 sleep(200)
13 elif button_b.is_pressed():
14 sleep(200)
15 display.scroll(microphone.sound_level())
Step 3: Improve it
- Add ‘show string’ blocks to make it clearer when temperature, sound and light readings are being displayed.
- You can modify the code to show temperature readings in Fahrenheit – see our Fahrenheit thermometer project for tips on how to do this.
- If you have the micro:bit V1, which doesn’t have a microphone, you can remove the code for button B and just take temperature and light level measurements.
This content is published under a Creative Commons Attribution-ShareAlike 4.0 International (CC BY-SA 4.0) licence.