The micro:bit Python Editor
Supporting students learning text-based coding with the BBC micro:bit
Why learn Python on the micro:bit?
Python is an excellent first text-based language to learn. Its instructions and syntax are based on natural language, making code easy to read, understand and modify.
As well as being widely used in education, it's used in industry, especially in the areas of data science and machine learning. Python is not just used by software developers, but also by people working in fields as diverse as medicine, physics and finance.
Python on the BBC micro:bit brings the benefits of physical computing to students aged 11-14, learning programming fundamentals through text-based coding: immersive, creative experiences for students that help build engagement and knowledge.
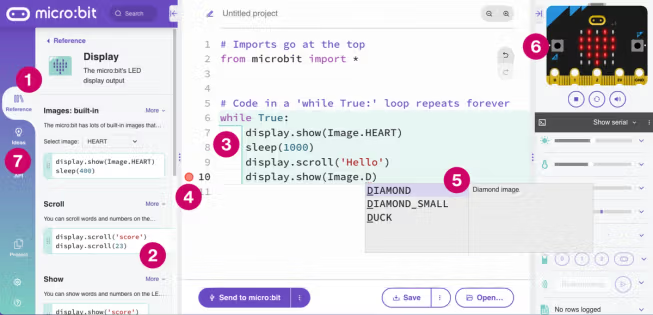
Features for education
Click on the headings below to discover some key features of the micro:bit Python Editor designed to overcome some common barriers, boost creativity and make the most of your coding time in class:
1. Reference
1. Reference
2. Drag and drop code snippets
2. Drag and drop code snippets
3. Code structure highlighting
3. Code structure highlighting
4. Error highlighting
4. Error highlighting
5. Auto-complete
5. Auto-complete
6. Simulator
6. Simulator
7. Ideas
7. Ideas
Overcoming barriers to learning
Click on the headings below to explore how the micro:bit Python Editor is designed to overcome common barriers to engaging and making progress with text-based coding:
Fear of a blank screen
Fear of a blank screen
Discoverability for creativity
Discoverability for creativity
Keyboard skills and memory
Keyboard skills and memory
Aid iterative project design
Aid iterative project design
Understanding program structure
Understanding program structure
Supporting debugging
Supporting debugging
Making the most of precious time
Making the most of precious time
Technical requirements
You just need a web browser to load the editor. Once loaded, it will continue to work, even if your internet connection becomes unstable.
The editor will work on any recent Windows, Mac or Chromebook computer.
If you want to send your code direct to your micro:bit, without downloading it as a HEX file first, you'll need to use Microsoft Edge or Google Chrome browsers. Click on 'Send to micro:bit' and follow the instructions on screen.
Saving work
Students can save their work locally, or in shared storage, as HEX project files which can be copied direct to a micro:bit or re-loaded in the editor. Code can also be saved as Python text files which can also be reloaded in the editor, either by dragging and dropping or using the 'Open' button.
You can also use the micro:bit Python Editor in live coding lessons in person, or remotely, using micro:bit classroom. This lets you save a whole class's code as one Word document and also as a file to resume work on the same projects at a later date. It's totally free of charge and no logins or passwords are needed.
Support for localisation
Although Python itself is in English, learning to code in text-based languages is made more accessible when students can read explanations and use a code editor with an interface in their own language.
The micro:bit Python editor's buttons, Reference content and Ideas projects have been translated into several languages including French, Spanish, Chinese, Japanese and Korean.
Click on the cog icon and then select 'Language':
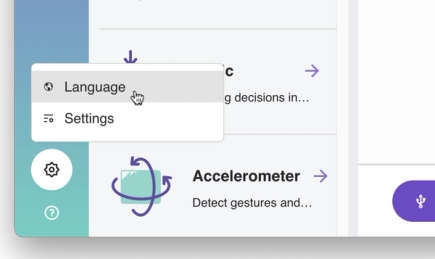
Find out more
You can find more detailed information and videos about how to use the micro:bit Python Editor on our support site.
Try it out
Test the editor for yourself right now. Browse the Reference and Ideas sections and see how quickly you and your students get inspired.
This short video will help you get coding in Python in seconds: