Step 1: Make it
What is it?
Recreate a classic toy from the 1950s with your micro:bit and customise it to make it your own.
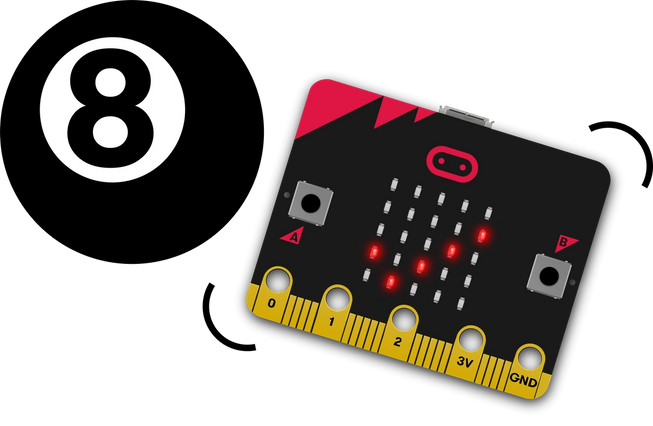
How it works
- A Magic 8-ball is a toy invented in the USA in the 1950s. Shaped like an oversized pool ball, you ask it a question like ‘will I be rich and famous one day?’, shake the ball and one of 20 different answers randomly appear in a window. Answers can be positive, negative - or somewhere in between.
- This program recreates a Magic 8-ball using the micro:bit’s accelerometer, its ability to make random numbers and its LED display output to show a tick for yes, a cross for no or a ‘meh’ face for ‘not sure’.
- The program generates a random number between 1 and 3 then uses if… then… else… if… statements to make different symbols appear depending on the number. This is known as selection.
- If the number is 3, it shows a tick for ‘yes’. If the number is 2, it shows a cross for ‘no’.
- The program doesn’t need to check if the number is 1, because if it’s not 3 or 2, it must be 1, in which case it shows a ‘meh’ face for ‘not sure.’
What you need
- micro:bit (or MakeCode simulator)
- MakeCode or Python editor
- battery pack (optional)
- some questions to ask your micro:bit
Step 2: Code it
Step 3: Improve it
- Make the image vanish after a few seconds.
- Make the micro:bit show different cryptic answers when you shake it, instead of pictures. It could say ‘I am not sure’ or ‘That remains to be seen’.
- Here’s another way of making a Magic 8-ball using Python.
This content is published under a Creative Commons Attribution-ShareAlike 4.0 International (CC BY-SA 4.0) licence.