Step 1: Make it
What is it?
A group game of ‘hot potato’ using radio – or hot duck!
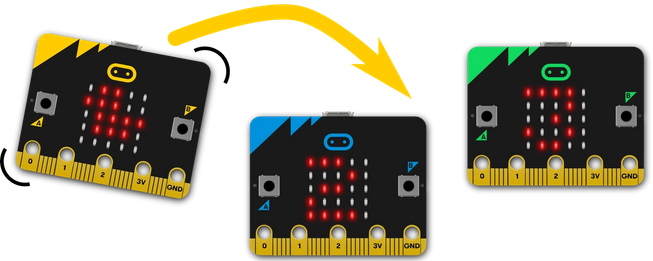
How it works
- Like the Teleporting duck game, this uses radio to send a ‘duck’ through the air between micro:bits. That game only works with 2 players as it sends the same message to everyone – soon you would find almost everyone had a duck and anyone could throw one.
- If you have more than 2 players each micro:bit needs to have a way of choosing which player will get the duck so each player’s program has a unique ID number, starting with 1.
- We store this in a variable called ID, and you’ll need to change this to 2, 3, 4 etc for each player before flashing the program onto their micro:bits.
- Set the players variable to the number of people to make sure everyone has a chance of getting the duck. The program shows the player ID number on the LED display at the start so you know who’s got which number.
- To make sure that only the player that has the duck can chuck it, the program only sends a message when you shake it if you have the duck. It keeps track of this using a Boolean variable called hasDuck. Boolean variables can only have two values: True or False. At the start only player 1 has the duck, no-one else can throw it.
- When player 1 shakes her micro:bit, the program generates a random number between 1 and the number of players. If the random number is not equal to her own ID number, it sends the new ID number by radio, clears her screen and sets her hasDuck variable to False.
- If the random number is equal to her own ID, she’ll need to throw again, but that’s better than the duck being sent to herself – and getting lost in hyperspace!
- If your micro:bit receives a number, it checks to see if it is equal to (matches) your ID number. If it does, congratulations, you now have the duck! A duck appears on your LED display, and your hasDuck variable gets set to True, meaning you can now chuck the duck to someone else.
- Please chuck ducks responsibly: make sure you don’t drop your micro:bit or hit a friend in your enjoyment of this game.
What you need
- 3 or more micro:bits
- MakeCode or Python editor
- battery packs (optional)
- a group of people to play with
Step 2: Code it
1from microbit import *
2import radio
3import random
4
5radio.config(group=42)
6players = 3
7ID = 3
8display.show(ID)
9if ID == 1:
10 hasDuck = True
11else:
12 hasDuck = False
13radio.on()
14
15while True:
16 message = radio.receive()
17 if accelerometer.was_gesture('shake'):
18 if hasDuck:
19 sendTo = random.randint(1, players)
20 if sendTo != ID:
21 display.clear()
22 radio.send(str(sendTo))
23 if message:
24 if message == str(ID):
25 hasDuck = True
26 display.show(Image.DUCK)
27 else:
28 hasDuck = False
29
Step 3: Improve it
- Change the program to send other things instead of ducks.
- At the moment, if it picks a random number that’s the same as your own ID number, you have to shake again. Modify the program so this never happens. There may be more than one way to do this.
- Player 1 always has the duck at the start of the game. Could you improve the program so the first player with the duck is chosen at random? How would you communicate this to every player’s micro:bit?
This content is published under a Creative Commons Attribution-ShareAlike 4.0 International (CC BY-SA 4.0) licence.