Step 1: Make it
What is it?
A radio-controlled remote alarm so when you know someone’s turned the lights on – or opened a drawer or bag.
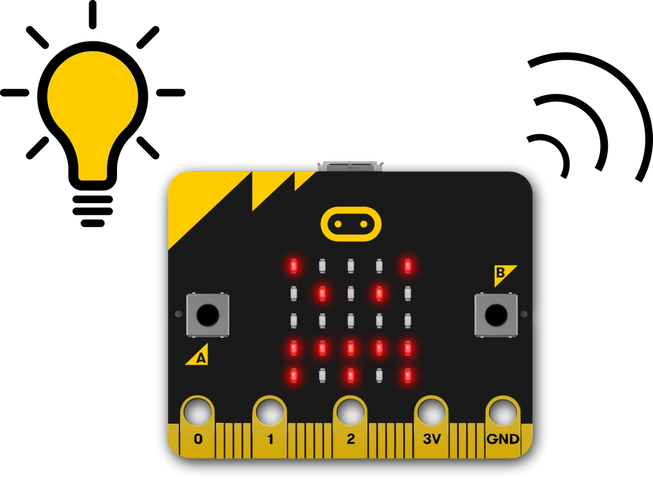
How it works
- Like the Sunlight sensor project, this uses the micro:bit’s LEDs as a light sensor to measure how much light is falling on the display.
- Using a loop, every 10 seconds the sensor program sends a radio message to say if it’s light or dark. It uses selection to transmit a ‘lights on’ message if the light measurement is more than (>) 50, and ‘lights off’ if it’s less than (<) 50. You may need to change these values depending on the lighting conditions where you are.
- Pressing input button A shows a light measurement on the LED display output which will help you set up your alarm, so you can change 50 to the best number for your environment.
- The alarm program reacts whenever it gets a message. If the message is equal to ‘lights off’ then it clears the LED display, but if it is equal to ‘lights on’ then it shows an angry face and plays the ‘BADDY’ alarm sound.
- Make sure your sensor and alarm use the same radio group – any number between 0 and 255.
What you need
- 2 micro:bits, at least one with a battery pack
- something precious to keep safe
- optional headphones, buzzers or powered speakers for alarm
Step 2: Code it
Sensor / transmitter:
Alarm / receiver:
Step 3: Improve it
- Combine this with the Tilt alarm project to sense when something is being moved OR if the lights go on.
- Make the alarm work the other way so it goes off if someone turns the lights off.
- Adapt it to monitor energy use: use a variable to track the number of seconds the lights are left on or trigger an alarm only after they’ve been on too long.
This content is published under a Creative Commons Attribution-ShareAlike 4.0 International (CC BY-SA 4.0) licence.